Python CGI Programming
Until now we did a lot of stuffs with programming that are not related to web or network. Now it’s time for CGI. As the name suggests, CGI means “Common” gateway interface for everything. CGI is one of the important parts of HTTP (Hyper-Text Transfer Protocol).
It is a set of standards that defines a standard way of passing information or web-user request to an application program & to get data back to forward it to users. This is the exchange of information between web-server & a custom script. When the users requested the web-page the server sends the requested web-page. The web server usually pass the information to all application programs that processes data and sends back an acknowledged message; and this technique of passing data back-&-forth between server & application is the Common Gateway Interface. The current version of CGI is CGI/1.1 & CGI/1.2 is under process.
Browsing
What happens when a user clicks a hyperlink to browse a particular web-page or URL (Uniform Resource Locator).
The steps are:
It may become possible to set-up an HTTP server because when a certain directory is requested that file is not sent back; instead it is executed as a program and that program’s output is displayed back to your browser.
Configuring CGI
The steps are:
The architecture of CHI is shown below:
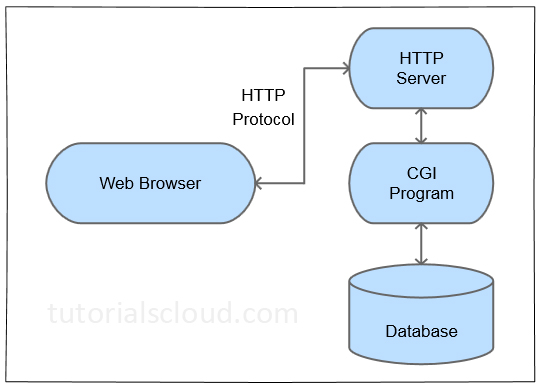
Python CGI Program Structure
The output of Python CGI script must consist of two sections separated by a blank line. The first part contains the number of headers that describes the client what kind of data is following.
Python code header section looks something like this:
Example:
#!/usr/bin/python
print "Content-Type : text/html"
# then comes the rest hyper-text documents
print "<html>"
print "<head>"
print "<title>My First CGI-Program </title>"
print "<head>"
print "<body>"
print "<h3>This is HTML's Body section </h3>"
print "</body>"
print '</html>'
Save this file as CGI.py. When you will open that saved file, the output becomes:
Output:
This is HTML's Body section
This is a simple Python script that writes its output on STDOUT file i.e. on screen.
Use Of CGI Module
We programmers write CGI scripts in Python, they can add these lines:
import cgitb
cgitb.enable()
The above code triggers special exception handler that will display detailed report in the web-browser in case of occurrence of any error.
HTTP Header
Few are the important lists of HTTP header frequently used in CGI programs. These are:
HTTP header | Value |
---|---|
Content-type | text/html |
Expires | Date |
Location | URL |
Set-Cookie | String |
Content-length | N |
CGI Environment Variables
Environment Variables | Description |
---|---|
CONTENT_TYPE | describes the data-type of the content |
HTTP_COOKIE | returns the visitor’s cookie, if one is set |
CONTENT_LENGTH | It is available for POST request to define the length of query information |
HTTP_USER_AGENT | defines the browser type of the visitor |
PATH_INFO | defines the path of CGI script |
REMOTE_HOST | defines the host-name of the visitor |
REMOTE_ADDR | defines the IP Address of the visitor |
REQUEST_METHOD | used to make request & the most common methods are – GET and POST |
Comments
Post a Comment